|
| 1 | +[](https://github.com/Jim-Hodapp-Coaching/esp32-wroom-rp/actions/workflows/build_and_test.yml) |
| 2 | + |
| 3 | +# esp32-wroom-rp |
| 4 | +A Rust-based RP2040 series driver providing WiFi functionality via Espressif ESP32-WROOM-32U/UE WiFi daughter controllers/boards. |
| 5 | + |
| 6 | +Supports the [ESP32-WROOM-32E](https://www.espressif.com/sites/default/files/documentation/esp32-wroom-32e_esp32-wroom-32ue_datasheet_en.pdf), [ESP32-WROOM-32UE](https://www.espressif.com/sites/default/files/documentation/esp32-wroom-32e_esp32-wroom-32ue_datasheet_en.pdf) modules. |
| 7 | + |
| 8 | +Future implementations will support the [ESP32-WROOM-DA](https://www.espressif.com/sites/default/files/documentation/esp32-wroom-da_datasheet_en.pdf) module. |
| 9 | + |
| 10 | +## Usage |
| 11 | + |
| 12 | +```rust |
| 13 | +use rp2040_hal as hal; |
| 14 | + |
| 15 | +use esp32_wroom_rp::{wifi::Wifi, gpio::EspControlPins}; |
| 16 | +use embedded_hal::blocking::delay::DelayMs; |
| 17 | + |
| 18 | +use embedded_hal::spi::MODE_0; |
| 19 | +use fugit::RateExtU32; |
| 20 | +use hal::{clocks::Clock, pac}; |
| 21 | + |
| 22 | +let _spi_miso = pins.gpio16.into_mode::<hal::gpio::FunctionSpi>(); |
| 23 | +let _spi_sclk = pins.gpio18.into_mode::<hal::gpio::FunctionSpi>(); |
| 24 | +let _spi_mosi = pins.gpio19.into_mode::<hal::gpio::FunctionSpi>(); |
| 25 | + |
| 26 | +let spi = hal::Spi::<_, _, 8>::new(pac.SPI0); |
| 27 | + |
| 28 | +// Exchange the uninitialized SPI driver for an initialized one |
| 29 | +let spi = spi.init( |
| 30 | + &mut pac.RESETS, |
| 31 | + clocks.peripheral_clock.freq(), |
| 32 | + 8_000_000u32.Hz(), |
| 33 | + &MODE_0, |
| 34 | +); |
| 35 | + |
| 36 | +let esp_pins = EspControlPins { |
| 37 | + // CS on pin x (GPIO7) |
| 38 | + cs: pins.gpio7.into_mode::<hal::gpio::PushPullOutput>(), |
| 39 | + // GPIO0 on pin x (GPIO2) |
| 40 | + gpio0: pins.gpio2.into_mode::<hal::gpio::PushPullOutput>(), |
| 41 | + // RESETn on pin x (GPIO11) |
| 42 | + resetn: pins.gpio11.into_mode::<hal::gpio::PushPullOutput>(), |
| 43 | + // ACK on pin x (GPIO10) |
| 44 | + ack: pins.gpio10.into_mode::<hal::gpio::FloatingInput>(), |
| 45 | +}; |
| 46 | + |
| 47 | +let wifi = Wifi::init(spi, esp_pins, &mut delay).unwrap(); |
| 48 | +let version = wifi.firmware_version(); |
| 49 | +``` |
| 50 | + |
| 51 | +## Hardware |
| 52 | + |
| 53 | +In order to run this code you need to purchase some hardware. This section provides a list of required hardware |
| 54 | +needed at minimum, and some suggested items to make your life even easier. |
| 55 | + |
| 56 | +### Required Hardware |
| 57 | + |
| 58 | +1. [Raspberry Pi Pico with pre-soldered headers](https://www.elektor.com/raspberry-pi-pico-rp2040-with-pre-soldered-headers) (2x) |
| 59 | + * [Alternate distributors](https://www.raspberrypi.com/products/raspberry-pi-pico/) |
| 60 | + |
| 61 | +2. Pimoroni Pico Wireless Pack (1x) |
| 62 | + * [US distributor](https://www.digikey.com/en/products/detail/pimoroni-ltd/PIM548/15851367) |
| 63 | + * [UK distributor](https://shop.pimoroni.com/products/pico-wireless-pack?variant=32369508581459) |
| 64 | + * [EU distributor](https://www.elektor.com/pimoroni-raspberry-pi-pico-wireless-pack) |
| 65 | + |
| 66 | +3. [Breadboard](https://www.sparkfun.com/products/12614) (1x) |
| 67 | + * __Note__: If you already have a medium/large breadboard, then don't worry about purchasing this specific one |
| 68 | + |
| 69 | + |
| 70 | +### Optional but Helpful Hardware |
| 71 | + |
| 72 | +1. [Break Away Headers](https://www.sparkfun.com/products/116) (1x) |
| 73 | + * If you want to solder headers to the non-pre-soldered BME280 sensor board from #2 above |
| 74 | + |
| 75 | +2. [Multi-length Jumper Wire Kit 140pcs](https://www.sparkfun.com/products/124) (1x) |
| 76 | + |
| 77 | +3. [Straight 7" Jumper Wires M/M](https://www.sparkfun.com/products/11026) (1x) |
| 78 | + * Helpful to have some of these on hand |
| 79 | + |
| 80 | +4. [Straight 6" Jumper Wires M/F](https://www.sparkfun.com/products/12794) (1x) |
| 81 | + * Helpful to have some of these on hand |
| 82 | + |
| 83 | +5. [Saleae Logic 8](https://www.saleae.com/) (1x) |
| 84 | + * __Note__: Only needed if you'd like to participate in developing/debugging parts of this project that communicate |
| 85 | + on the SPI/I2C buses |
| 86 | + |
| 87 | +### Wiring Details |
| 88 | + |
| 89 | +Start with the section [Pico to Pico Wiring in this article](https://reltech.substack.com/p/getting-started-with-rust-on-a-raspberry?s=w) to set up using two Picos together, one as a Picoprobe (flash/debug) and the other as your embedded target. |
| 90 | + |
| 91 | +Once properly wired, it should look similar to the following: |
| 92 | + |
| 93 | +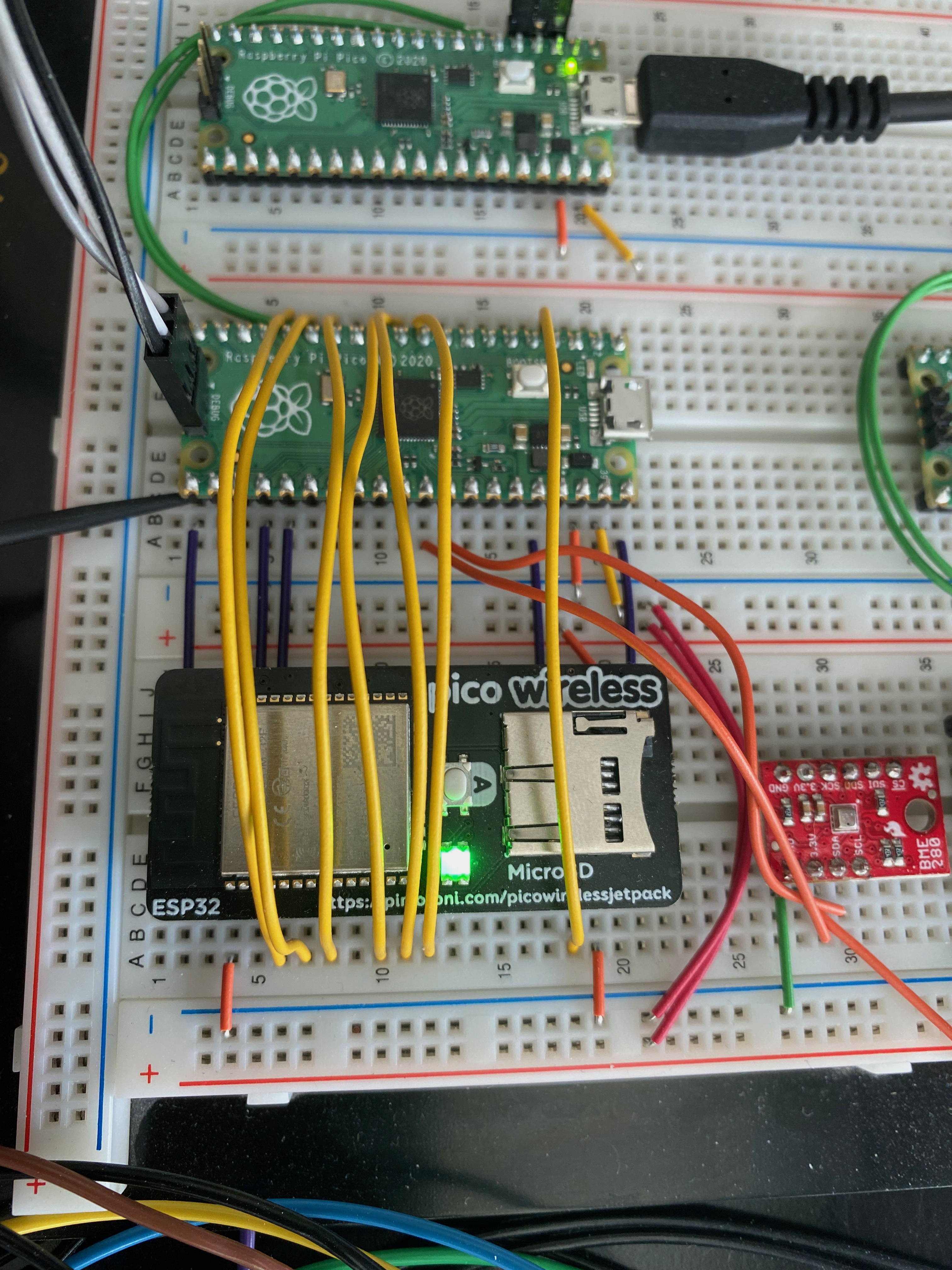 |
| 94 | + |
| 95 | +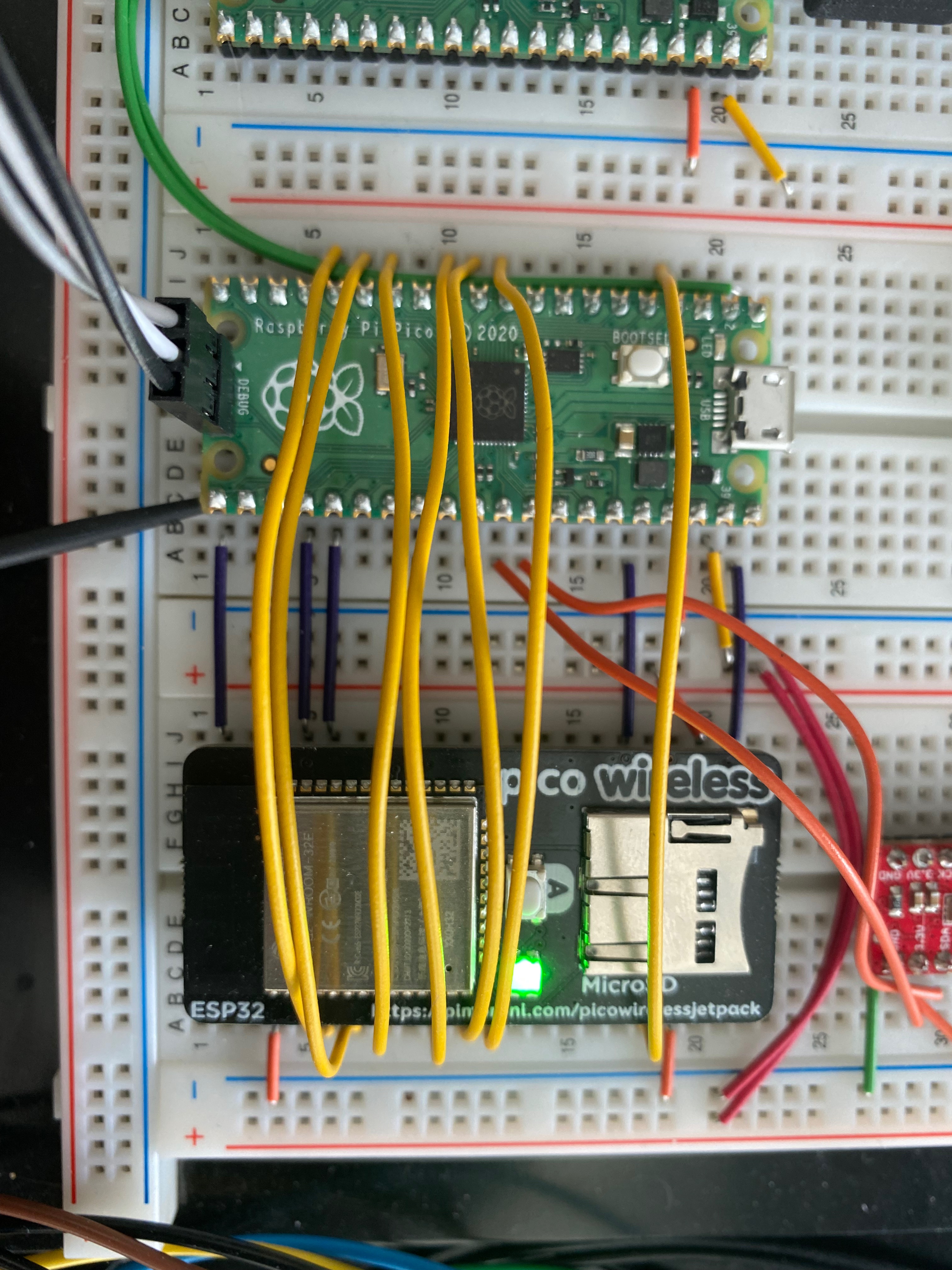 |
| 96 | + |
| 97 | +__Pico to ESP32 WiFi__ |
| 98 | + |
| 99 | +The following table lists the pin name and pin number to properly wire between a Pico board and an ESP32 WiFi. This can be done on a breadboard such as the one listed above. Note that V+/- rail means the +/- columns on the breadboard for use as +5 VDC and GND respectively. |
| 100 | + |
| 101 | +| Pico | ESP32 WiFi | Adafuit Airlift | Breadboard | |
| 102 | +| ----------------- | ---------------- | ----------------| ---------- | |
| 103 | +| | GND (Pin 3) | GND (Pin 3) | V- rail | |
| 104 | +| GP2 (Pin 4) | GPIO0 (Pin 4) | GP0 (Pin 10) | | |
| 105 | +| GP7 (Pin 10) | ESP_CSn (Pin 10) | CS (Pin 7) | | |
| 106 | +| GP8 (Pin 11) | | | | |
| 107 | +| GP9 (Pin 12) | | | | |
| 108 | +| GP10 (Pin 14) | ACK (Pin 14) | Busy (Pin 8) | | |
| 109 | +| GP11 (Pin 15) | RESETn (Pin 15) | RSTn (Pin 9) | | |
| 110 | +| GP12 (Pin 16) | SW_A (Pin 16) | N/A | | |
| 111 | +| | GND (Pin 18) | | V- rail | |
| 112 | +| VBUS (Pin 40) | VBUS (Pin 40) | | | |
| 113 | +| VSYS (Pin 39) | VSYS (Pin 39) | VIN (Pin 1) | V+ rail | |
| 114 | +| GND (Pin 38) | GND (Pin 38) | | V- rail | |
| 115 | +| 3V3(OUT) (Pin 36) | 3V3 (Pin 36) | 3Vo (Pin 2) | | |
| 116 | +| GP19 (Pin 25) | MOSI (Pin 25) | MOSI (Pin 5) | | |
| 117 | +| GP18 (Pin 24) | SCLK (Pin 24) | SCK (Pin 4) | | |
| 118 | +| | GND (Pin 23) | | V- rail | |
| 119 | +| GP16 (Pin 21) | MISO (Pin 21) | MISO (Pin 5) | | |
| 120 | + |
| 121 | + |
| 122 | +*** |
| 123 | + |
| 124 | +## Software Requirements |
| 125 | +- The standard Rust tooling (cargo, rustup) which you can install from https://rustup.rs/ |
| 126 | + |
| 127 | +- Toolchain support for the cortex-m0+ processors in the rp2040 (thumbv6m-none-eabi) |
| 128 | + |
| 129 | +- flip-link - this allows you to detect stack-overflows on the first core, which is the only supported target for now. |
| 130 | + |
| 131 | +## Installation of development dependencies |
| 132 | +```sh |
| 133 | +rustup target install thumbv6m-none-eabi |
| 134 | +cargo install flip-link |
| 135 | +cargo install probe-run |
| 136 | +``` |
| 137 | + |
| 138 | +## Building the crate and running the examples |
| 139 | + |
| 140 | +To build the esp32-wroom-rp crate: |
| 141 | +```sh |
| 142 | +cargo build |
| 143 | +``` |
| 144 | + |
| 145 | +To build all examples |
| 146 | +```sh |
| 147 | +cd cross |
| 148 | +cargo build |
| 149 | +``` |
| 150 | + |
| 151 | +To build a specific example (e.g. get_fw_version): |
| 152 | +```sh |
| 153 | +cd cross |
| 154 | +cargo build --bin get_fw_version |
| 155 | +``` |
| 156 | + |
| 157 | +To run a specific example (e.g. get_fw_version): |
| 158 | +```sh |
| 159 | +cd cross |
| 160 | +cargo run --bin get_fw_version |
| 161 | +``` |
| 162 | + |
| 163 | +## Running the crate's unit tests |
| 164 | +```sh |
| 165 | +cargo test |
| 166 | +``` |
| 167 | + |
| 168 | +## Getting Involved |
| 169 | + |
| 170 | +This project launched in April, 2022). See the main page section [Getting Involved](https://github.com/Jim-Hodapp-Coaching#getting-involved) for more info on how to contribute to this project and the Rust Never Sleeps community. |
| 171 | + |
| 172 | +To get involved, please [request to join the community here on GitHub](https://rustneversleeps.wufoo.com/forms/z1x3dy1j0ycafxq/) and then start contributing to the [research and design discussions](https://github.com/Jim-Hodapp-Coaching/esp32-wroom-rp/discussions) currently underway. |
| 173 | + |
| 174 | +## Project Team |
| 175 | + |
| 176 | +* Architect: [Caleb Bourg](https://github.com/calebbourg) |
| 177 | +* Rust Developer: [Dilyn Corner](https://github.com/dilyn-corner) |
| 178 | +* Rust Developer: [Glyn Matthews](https://github.com/glynos) |
| 179 | +* Project Oversight & Rust Developer: [Jim Hodapp](https://github.com/jhodapp) |
0 commit comments